TLMBoy: The Audio Processing Unit (APU) - Noise Channel
In this part of my Game Boy simulator post series, I will cover the details of the noise channel of the so-called Audio Processing Unit (APU). Unlike modern hardware, the Game Boy cannot (or is not supposed) to play black sample-based audio recordings. Rather the APU has 4 different channels that act like instruments controlled by notes and dynamics. One of these channels is the noise channel. It’s quite versatile and can be used to resemble snares, hi-hats, explosions, or even waves washing up on shore.
When it comes to information about the Game Boy’s hardware, there’s already plenty information available. The following sources helped me a lot to write this post and my Game Boy simulator:
Official Game Boy Programming Manual
Game Boy Development Wiki
Game Boy CPU Manual
Pan Docs (my favorite source)
To not write yet another technical documentation like the sources above, this post follows a more example-driven approach. So, rather than getting lost in every tiny obscure behavior, I first highlight the general principles of the noise channel, which is then followed by some practical examples on how games made use of it. I also provide a test ROM, which can be used for testing in emulator/simulator development.
Contents
Overview
Similar to other units of the Game Boy (DMA, Pixel Processing Unit, etc.), communication with the APU is facilitated by memory-mapped I/O. That means if you want to tell the APU something you just write something into certain memory-mapped registers, while information about the APU’s current status can retrieved by reading these registers. For the noise channel, the following 4 registers are relevant:
Name | Address | Bits | Function |
---|---|---|---|
NR41 | 0xFF20 | --LL LLLL |
Length load |
NR42 | 0xFF21 | VVVV APPP |
Starting volume, envelope mode, period |
NR43 | 0xFF22 | SSSS WDDD |
Clock shift, width mode of LFSR, divisor code |
NR44 | 0xFF23 | TL-- ---- |
Trigger, length enable |
In the following these registers are described in greater detail.
NR41: Length Timer
This register is used to control the length of a sound. In musical terms, this refers to a note’s duration. The register has only one field, controlling the length of a sound as follows:
[0:5] 6-bit length load: Can be read from or written to. The 6 bits are interpreted as an unsigned number ranging from 0 to 63. This number determines the length of the sound: length = (64-value)*(1/256) seconds. So, the shortest sound is 1/256 second, while the longest is 1/4 second. Note that the “64-value” part leads to some counterintuitive behavior. When writing 0, you get the longest possible length, an when writing 63, you get the shortest possible length. If you want indefinite sustain, disable Bit 6 in register NR44.
NR42: Envelope
The envelope register is used to control the volume envelope of a sound. The volume envelope describes how the volume of a sound changes over time. For example, a decreasing envelope can be used to mimic some kind of decay as with pianos or guitars. The register comprises 3 fields:
[0:2] 3-bit envelope period:
Interpreted as a 3-bit unsigned integer (0-7).
Determines how often the envelope is updated.
A decrement/increment happens every period*(1/64) second.
Writing 0 disables the envelope.
[3:3] 1-bit envelope add mode:
0 → decrement volume, 1 → increment volume.
[4:7] 4-bit starting volume of the envelope:
Represents a starting volume between 0-15.
The value is incremented/decremented depending on the envelope mode and period.
Important: You can read these bits but the hardware does not update them!
NR43: Noise Shape
This register is used to control the noises’s shape/color. Depending on the settings you can create everything from white noise to high-pitched metallic sounds. To create pseudo-random numbers for the noise channel, the Game Boy uses a Linear-Feedback Shift Register (LFSR):
Each time the LFSR is ticked, it performs the following three steps:
- The low two bits (0 and 1) are XORed and negated.
- The result of the XOR is put into the now-empty high bit (either Bit 15 or Bit 7 depending on the mode).
- All bits are shifted right by one.
The frequency by which new values are generated is: 4.194304 MHz / ( divider « shift), whereby divider is in {8, 16, 32, 48, 64, 80, 96, 112}, and shift is between 0 and 13. Hence, the highest frequency is 524,288 MHz and the lowest frequency is 4.57 Hz. Divider, width mode, and clock shift are derived from the following fields:
[0:2] 3-bit divider: Interpreted as a 3-bit unsigned integer (0-7). See formula.
[3:3] 1-bit width mode: Width of the LFSR. 0 → 15 bit, 1 → 7 bit.
[4:7] 4-bit clock shift: Interpreted as a 4-bit unsigned integer (0-15). See formula.
According to the programmer manual, the values 14 and 15 are illegal. Interestingly, this constraint is not mentioned in all documentation you can find online.
The LFSR has some interesting properties that I want to highlight in greater detail. For instance, in 7-bit mode, the generated pattern repeats every 127 cycles. In case you want to see it yourself, use this python script:
#!/usr/bin/python
IND = 7
NUM_SAMPLES = 128
reg = 0
print("Cycle\tLFSR\tWaveform Output")
for i in range(NUM_SAMPLES):
val = not ((reg & 1) ^ ((reg >> 1) & 1))
reg |= (val << IND)
reg >>= 1
output = reg & 1
print(f"{i}\t", "{0:07b}\t".format(reg), output)
It generates the following table:
Cycle | Value | Waveform Output |
---|---|---|
0 | 01000000 | 0 |
1 | 01100000 | 0 |
2 | 01110000 | 0 |
3 | 01111000 | 0 |
4 | 01111100 | 0 |
5 | 01111110 | 0 |
6 | 00111111 | 1 |
7 | 01011111 | 1 |
… | … | … |
127 | 01000000 | 0 |
Interestingly, the only value a 7-bit LFSR never reaches is all bits being 1. This is for a good reason, as such a value would lock the LFSR permanently in this state, resulting in only 1s being generated for the output. In practice it’s actually possible to arrive in such a situation, when switching from 15-bit mode to 7-bit in the right moment.
NR44: Channel Control
The channel control register only comprises two 1-bit fields:
[6:6] 1-bit length enable:
0 → Regardless of the length data in NR41 sound can be produced consecutively.
1 → Sound is generated during the time period set by the length data in NR41.
After this period the sound 4 ON flag (bit 3 of NR52) is reset.
[7:7] 1-bit trigger (write-only): Writing 1 to this bit causes the following things:
The noise channel is enabled. If the length timer expires it is reset. Envelope timer is reset.
Volume is set to contents of NR42 initial volume. LFSR bits are reset.
Noise Simulator
After all these technical details, it’s time for some practical evaluation. To get a better understanding of how the individual register and their fields are playing together in practice, I wrote a Javascript-based noise simulator. In the table below you can setup individual fields and listen to the sound they would create on the Game Boy. Note that the simulator repeats every 2 seconds. Predefined setups of some games are provided in the next section.
Register | Setting |
---|---|
NR41: Length Timer | Length |
NR42: Envelope |
Volume
Envelope mode Envelope period |
NR43: Noise Shape |
Divisor
Shift LFSR Width Resulting LFSR Sample Rate: 524288 Hz |
NR44: Channel Control |
Length enable:
|
Since only having this in Javascript is lame, I also wrote the same application for the Game Boy:
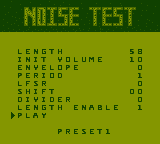
The source code and the corresponding ROM can be found in this Github repository.
Examples
To see the noise channel in action, I tried to find out how different games make use of this channel. Here’s what I found.
Tetris
In the title theme of Tetris, I found the following two settings:
Tetris Hi-hat:
NR41: 0011 1010 -> 58 -> length = 1/42 s (~23 ms)
NR42: 1010 0001 -> Envelope: Decrement every 1/64s (~16ms), starting from volume 10
NR43: 0000 0000 -> 15-bit LFSR, divisor 8, shift 0 (524,288 Hz)
NR44: 1100 0000 -> Sound according to length
Tetris Snare:
NR41: 0010 1001 -> 41 -> length = 23/264 s (~90 ms)
NR42: 1011 0001 -> Envelope: Decrement every 1/64s (~16ms), starting from volume 11
NR43: 0000 0001 -> 15-bit LFSR, divisor 16, shift 0 (262,144 Hz)
NR44: 1100 0000 -> Sound according to length
The first setting is used for something hi-hat-like sound, while the other one is used for a snare. As you can see and hear, they are not too different. The snare has a slightly higher starting volume, a greater length, and uses a higher divisor. All of that doesn’t really change the color of the sound but makes the snare more dominant compared to the hi-hat.
Super Mario Land
When defeating a Bombshell Koopa in Super Mario Land, the noise channel is used to create a sound of an explosion.
Bombshell Koopa Explosion:
NR41: 0000 0000 -> 0 -> length = 64/256 s (250 ms)
NR42: 1111 0100 -> Envelope: Decrement every 4/64s (~62.5ms), starting from volume 15
NR43: 0101 0111 -> 15-bit LFSR, divisor 112, shift 5 (1170.3 Hz)
NR44: 1000 0000 -> Sound not according to length
In comparison to the settings in Tetris, Super Mario Land does not use the length register, as this would limit the sound to 250 ms at most. By only relying on the decrement register, it takes roughly 1 second for the sound to go from volume 15 to volume 0. With an LFSR sample rate of 1170.29 Hz, the noise is also very chiptune-like.
So far, all examples used a 15-bit LFSR. This is not very surprising as 7-bit provides very little randomness. In fact, the 127-cycle repetition gives it a metallic high-pitched sound for higher LFSR sample rates, which is very far away from being white noise. This can be heard in parts of the sound that are played when defeating a Fighter Fly:
Fighter Fly Defeated:
NR41: 0000 0000 -> 0 -> length = 64/256 s (250 ms)
NR42: 0010 1100 -> Envelope: Increase every 4/64s (~62.5 ms), starting from volume 2
NR43: 0001 1110 -> 7-bit LFSR, divisor 96, shift 1 (21,845.3 Hz)
NR44: 1000 0000 -> Sound not according to length
Note that this only a part of the sound when defeating a Fighter Fly. Some of the registers are altered after a short period of time to make the sound more insect-like.
Bomberman GB
For lower LFSR sample rates, the sound of a 7-bit LFSR gets “noisier” and somewhat approximates the sound of a 15-bit LFSR. Nevertheless, the 127-cycle repetition leads to some kind of reverb effect. The exploding bombs in Bomberman GB are a good example of an explosion sound with a touch of reverb.
Bomb explosion:
NR41: 1111 0111 -> 0 -> length = 55/256 s (214 ms)
NR42: 1110 0101 -> Envelope: Decrease every 5/64s (~78.1 ms), starting from volume 14
NR43: 0110 1011 -> 7-bit LFSR, divisor 48, shift 6 (87,381.3 Hz)
NR44: 1000 0000 -> Sound not according to length
The Legend Of Zelda: Link’s Awakening
Another example showcasing the great versatility of the noise channel can be found in the intro of The Legend Of Zelda: Link’s Awakening. Here, a fading white noise sound is used to mimic the waves washing up on shore.
Wave fading in:
NR41: 0000 0000 -> 0 -> length = 64/256 s (250 ms)
NR42: 0000 1111 -> Envelope: Increase every 7/64s (~109.4 ms), starting from volume 0
NR43: 0011 0000 -> 15-bit LFSR, divisor 8, shift 3 (65,536 Hz)
NR44: 1000 0000 -> Sound not according to length
Wave fading out:
NR41: 0000 0000 -> 0 -> length = 64/256 s (250 ms)
NR42: 0110 0111 -> Envelope: Decrease every 7/64s (~109.4 ms), starting from volume 6
NR43: 0000 0011 -> 15-bit LFSR, divisor 48, shift 0 (87,381.3 Hz)
NR44: 1000 0000 -> Sound not according to length