TLMBoy: The Audio Processing Unit (APU) - Square Channel
In this part of my Game Boy simulator post series, I will cover the details of the square channel of the so-called Audio Processing Unit (APU). Unlike modern hardware, the Game Boy cannot (or is not supposed) to play black sample-based audio recordings. Rather the APU has 4 different channels that act like instruments controlled by notes and dynamics. Two of these channels are square channels, which are the focus of this post. These channels generate square waves at given frequencies allowing you to play notes just like an instrument. The first square channels also has some extra frequencies-shifting features, which can be used to create various kinds of sounds.
When it comes to information about the Game Boy’s hardware, there’s already plenty information available. The following sources helped me a lot to write this post and my Game Boy simulator:
Official Game Boy Programming Manual
Game Boy Development Wiki
Game Boy CPU Manual
Pan Docs (my favorite source)
Unlike the technical documentation from above, this post follows a more example-driven approach. So, rather than getting lost in every tiny obscure behavior, I first highlight the general principles of the square channel, which is then followed by some practical examples on how games made use of it. I also provide a test ROM, which can be used for testing in emulator/simulator development.
Contents
Overview
Similar to other units of the Game Boy (DMA, Pixel Processing Unit, etc.), communication with the APU is facilitated by memory-mapped I/O. That means if you want to tell the APU something you just write something into certain memory-mapped registers, while information about the APU’s current status can retrieved by reading these registers. For the two square channels, the following registers are relevant:
Square 1:
Name | Address | Bits | Function |
---|---|---|---|
NR10 | 0xFF10 | -PPP NSSS |
Sweep period, negate, shift |
NR11 | 0xFF11 | DDLL LLLL |
Wave duty, length load |
NR12 | 0xFF12 | VVVV EPPP |
Init volume, envelope mode, envelope period |
NR13 | 0xFF13 | FFFF FFFF |
Frequency LSB |
NR14 | 0xFF14 | TL-- -FFF |
Trigger, length enable, frequency MSB |
Square 2:
Name | Address | Bits | Function |
---|---|---|---|
NR21 | 0xFF16 | DDLL LLLL |
Wave duty, length load |
NR22 | 0xFF17 | VVVV EPPP |
Init volume, envelope mode, envelope period |
NR23 | 0xFF18 | FFFF FFFF |
Frequency LSB |
NR24 | 0xFF19 | TL-- -FFF |
Trigger, length enable, frequency MSB |
Since Square 2 has a subset of the features of Square 1, the following only highlights the details of Square 2. Except for the missing features (sweep period, negate, shift), Square 1 and Square 2 work similarly.
Square Channels
The square wave channel allows you to play - big surprise - square waves. This kind of wave truly defines the Game Boy’s chiptune sound. And since this channel is so important, the Game Boy allows you two play two square waves at the same time! While many synthesizers allow you to heavily modify the square wave, the Game Boy’s options are very limited in that regard. Anyway, first the very technical definition of the square wave channel register before we are heading to some examples.
NR10: Channel Sweep
The sweep channel can be used to change the frequency of the square wave over time. This is primarily used to model sound effects, such as hopping on a Goomba in Super Mario Land.
[0:2] 3-bit step: Each iteration, a new frequency is calculated as: F(i+1) = F(i) + F(i) / 2^step.
This value is also written back to register NR13 and NR14!
[3:3] 1-bit direction: 0 → frequency increase, 1 → frequency decrease.
[4:6] 3-bit period: The sweep is updated every (period * 7.8 milliseconds). A value of 0 disables the sweep.
NR11: Channel Length Timer & Duty
[0:5] 6-bit initial length timer:
Can be read from or written to.
The 6 bits are interpreted as an unsigned number ranging from 0 to 63.
This number determines the length of the sound: length = (64-value)*(1/256) seconds.
So, the shortest sound is 1/256 second, while the longest is 1/4 second.
Note that the “64-value” part leads to some counterintuitive behavior.
When writing 0, you get the longest possible length, an when writing 63, you get the shortest possible length.
If you want indefinite sustain, disable Bit 6 in register NR44.
[6:7] 2-bit duty cycle: Determines the duty cycles of the square wave (00 → 12.5%, 01 → 25%, 10 → 50%, 11 → 75%).
Note that 25% and 75% give the same audible impression when the square wave is played without the other channels.
NR12: Channel Volume & Envelope
[0:2] 3-bit Envelope update period: The envelope ticks at 64 Hz, and the channel’s volume is updated every Nth (given by 3-bit value) tick.
So, the fastest possible update is 64 Hz, while the slowest 8Hz. 0 disables the envelope.
[3:3] 1-bit envelope mode: 0 → decrement volume, 1 → increment volume.
[4:7] 4-bit initial volume: Starting volume representing values between 0-15. You can read these bits but the hardware does not update them!
NR13: Frequency LSB
[0:7] 8-bit frequency lower bits: The frequency comprises 11 bits in total (see NR14). The square channel uses a non-exposed, 11-bit counter that increases every time it is clocked. After 2047 it overflows, generates a signal, and is set to the value of NR13 and NR14. The resulting frequency is: 131,072/(2048-frequency). Hence, the lowest frequency is 64 Hz and highest ones is 131,072 Hz, which is already far out of the reach humans can hear.
NR14: Channel Control & Frequency MSB
[0:2] 3-bit frequency lower bits: Upper bits of the period. See NR13.
[6:6] 1-bit length enable:
0 = Regardless of the length data in NR14 sound can be produced consecutively.
1 = Sound is generated during the time period set by the length data in NR14.
After this period the sound 1 ON flag (bit 0 of NR52) is reset.
[7:7] 1-bit trigger (write-only): Writing 1 to this bit causes the following things:
The square channel is enabled. If the length timer expired it is reset. Envelope timer is reset.
Volume is set to contents of NR41 initial volume. The period divider is set to the contents of NR13 and NR14. Sweep does things.
Square Simulator
Here’s a Javascript-based square channel simulator. In the table below you can setup individual fields and listen to the sound they would create on the Game Boy. Note that the simulator repeats every 2 seconds. Predefined setups of some games are provided in the next section.
Register | Setting |
---|---|
NR10: Channel Sweep |
Step
Direction Period |
NR11: Length Timer |
Length
Duty |
NR12: Envelope |
Volume
Envelope mode Envelope period |
NR13/NR14: Square Frequency | Frequency |
NR13: Channel Control |
Length enable:
|
Besides an implementation in Javascript, I also wrote the same application for the Game Boy:
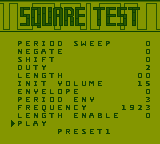
The source code and the corresponding ROM can be found in this Github repository.
Examples
In the following, examples of square channel in real-world software are provided. Click on “Use this setup” to load the square simulator with the corresponding setup.
Boot
One simple, yet iconic example of the square wave is the Game Boy’s boot sound. From examining the boot code (see also my boot ROM post), I found the two following register settings:
NR10: 0000 0000 -> No sweep
NR11: 1000 0000 -> Duty cycle 50%, length irrelevant due to NR14
NR12: 1111 0011 -> Full volume, decrement volume, update envelope every 3 envelope ticks
NR13: 1000 0011 -> Frequency: 1048.576 Hz (C6)
NR14: 1000 0111 -> Trigger, sound indefinite length, period upper 3 bits
NR10: 0000 0000 -> No sweep
NR11: 1000 0000 -> Duty cycle 50%, length irrelevant due to NR14
NR12: 1111 0011 -> Full volume, decrement volume, update envelope every 3 envelope ticks
NR13: 1100 0001 -> Frequency: 2080.50 Hz (C7)
NR14: 1000 0111 -> Trigger, sound indefinite length, period upper 3 bits
So, a very simple square with 50% duty and no fancy sweep settings. The sound starts at full volume and is then decremented every 3 envelope ticks. If I did the math correctly, that should correspond to a length of ~0.7s until the volume reaches 0. Note that the Game Boy plays two sounds to get this “bling bling.” First a C6, which is only played for four frames (~66 milliseconds), and then a C7, which is played for the full duration of ~0.7 seconds.
Super Mario Land
In Super Mario Land I found a few examples that make use the square’s sweep setting to model sound effects. Note that sound effects may involve multiple subsequent setting. In the following only single settings are provided.
When jumping on a Goomba, you get the following setting:
NR10: 0101 0111 -> step = 7, frequency increase, sweep period = 5
NR11: 1000 0000 -> duty = 2%, length = 0 (irrelevant due to NR14)
NR12: 0110 0010 -> envelope period = 2, decrement volume, volume = 6
NR13: 0000 0110 -> frequency = 1798
NR14: 1000 0111 -> trigger, sound indefinite length
Parts of the sound when taking a mushroom are very similar to the Goomba sound:
NR10: 0010 0111 -> sweep step = 7 , increase frequency, sweep period = 2
NR11: 1000 0000 -> length = 0, duty = 2
NR12: 0110 0010 -> envelope period = 2, decrement volume, volume = 6
NR13: 0111 0010 -> frequency = 1650
NR14: 1000 0110 -> trigger, sound indefinite length